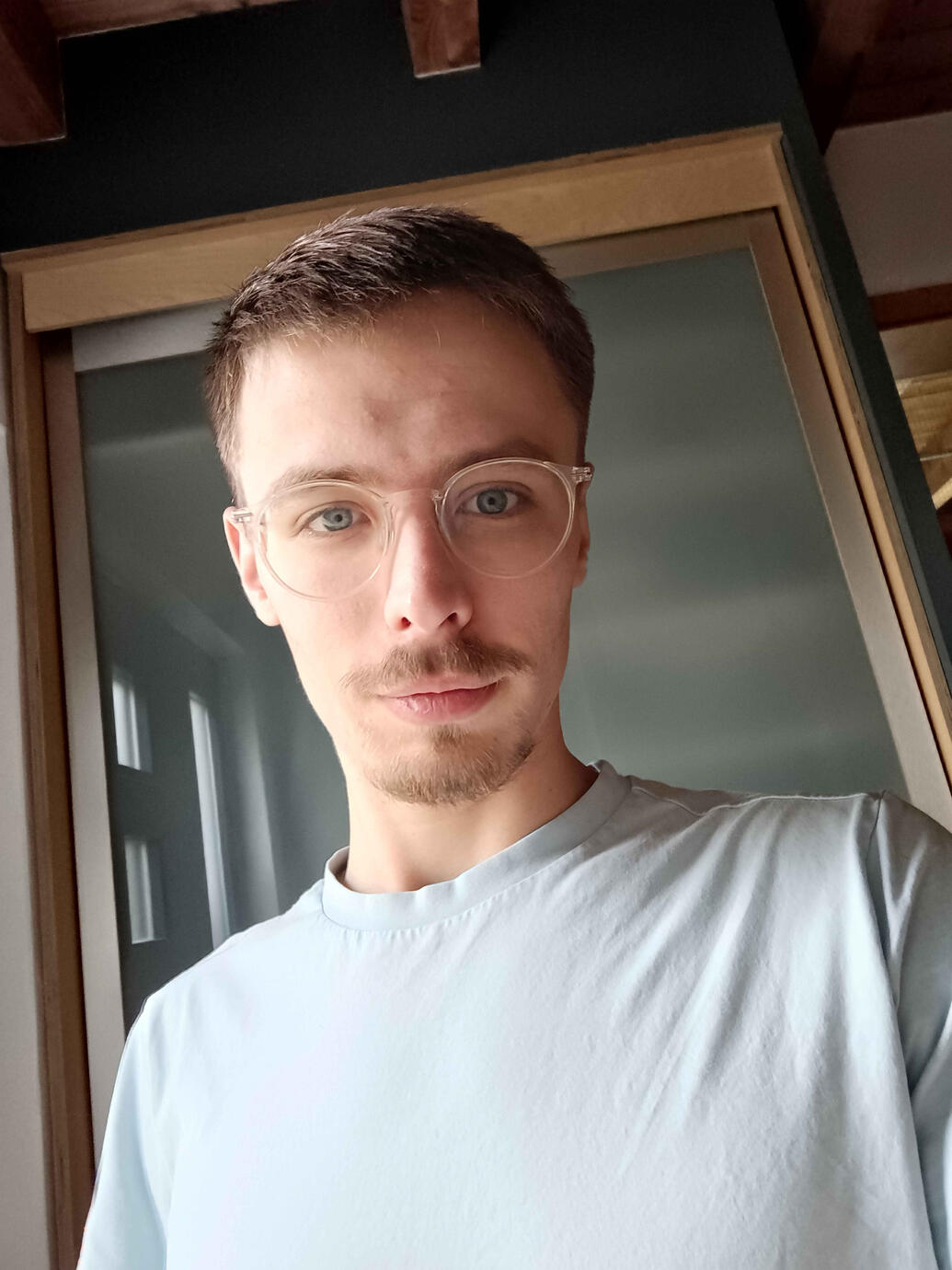
Thiel Leonhard
I'm Leo, a student at Mediadesign Hochschule München with a deep passion for programming and problem-solving. While my primary focus has been Game Engineering, my curiosity has led me to explore various aspects of computer science, with a growing emphasis on Cybersecurity.
Check out my website to see a bunch of cool games and projects I've been working on!
C++ Game Prototype
In this Project I tried to make an interesting approach to combine the Command Pattern with the Entity Component Pattern.
Game Idea
The game is built using C++ in conjunction with the SDL2 library.
The Player can
...move on a Grid (or perform actions)
...fight against opponents, who only perform an action when the player performs one
...undo the last action by pressing a button (and the penultimate action if the button is pressed repeatedly)
Implementing Software Design Patterns
Develop the foundational Entity Class.
Establish the base Component Class.
Implement dependencies, such as storing a vector of components within the Entity Class.
Define necessary Sub-Entities and Sub-Components.
Establish the Command Base Class.
Define the Command Subclasses.
Integrate the Command Pattern by including a Command Stack, within the Character Class.
Modify the script logic accordingly. (Actions are now executed through Commands, which are stored within the character's command stack)
Entities
Components
Commands
Gameplay
The game begins with the player in Move Mode. Here, the player is shown fields marked as up, down, left, and right. Clicking on these with the mouse moves the player accordingly. Once the player takes an action, the opponent must also take an action. Player actions include Moving, Attacking, and Defending, which can be switched using the keys W (Move/Walk), A (Attack), and D (Defend), respectively. The cell markings change accordingly.Opponents can only Move, Do Nothing, or Attack, but only after the player has taken an action. In combat, the player has the option to attack the opponent and accept a counterattack, or to block the opponent's attack, taking only half the damage and strengthening their next attack.At any time, the player can undo actions taken by themselves and all opponents, though the Attack Buff does not persist
Modes:
MoveMode
AttackMode (diagonally)
DefendMode
Implementing a custom GameManager to enhance the readability of the main.cpp file
The GameManager is responsible for managing all necessary tasks, including setup, event handling, and garbage collection.
Therefore, the main.cpp file can also look like this:
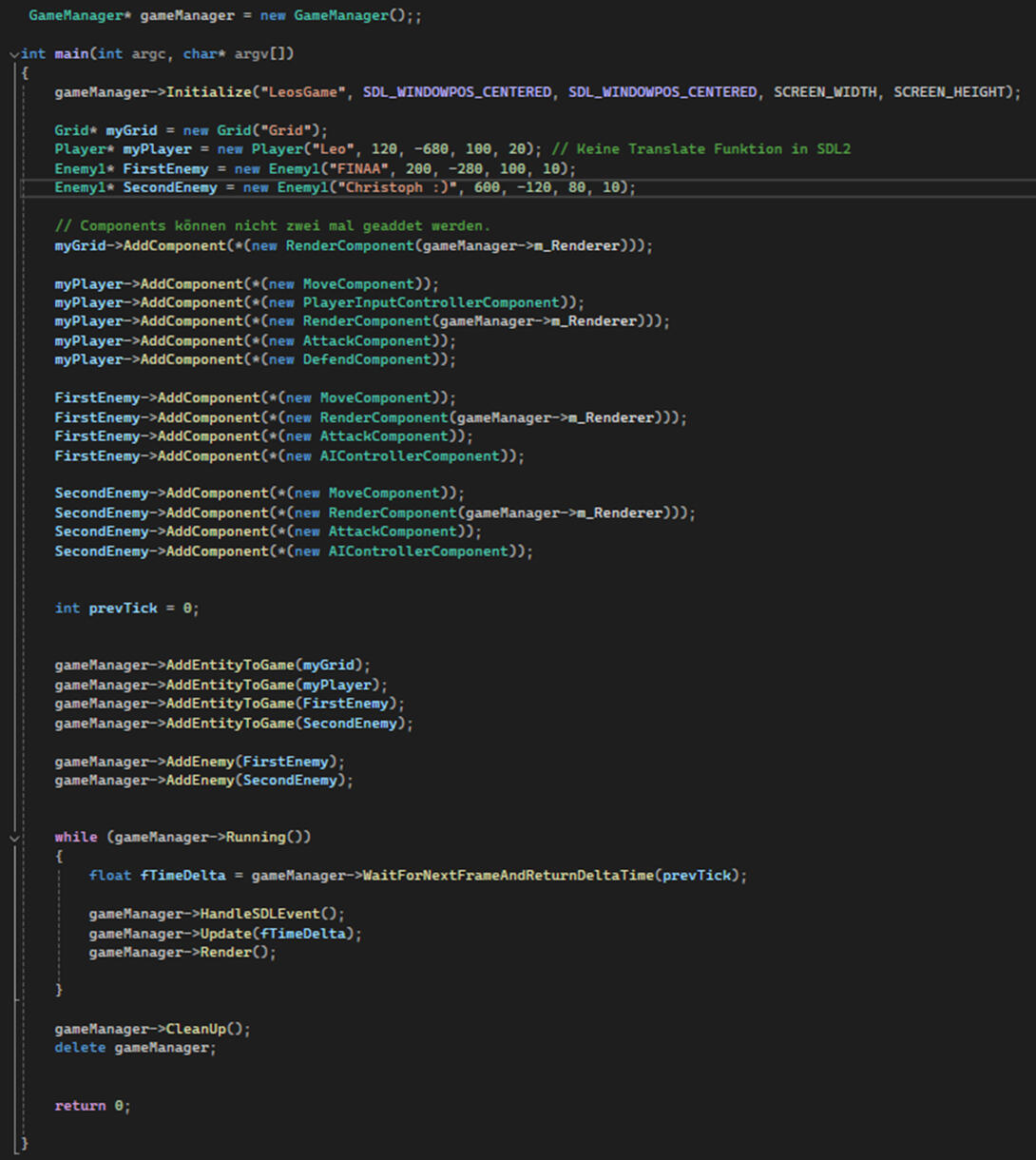
Complete Class Diagram
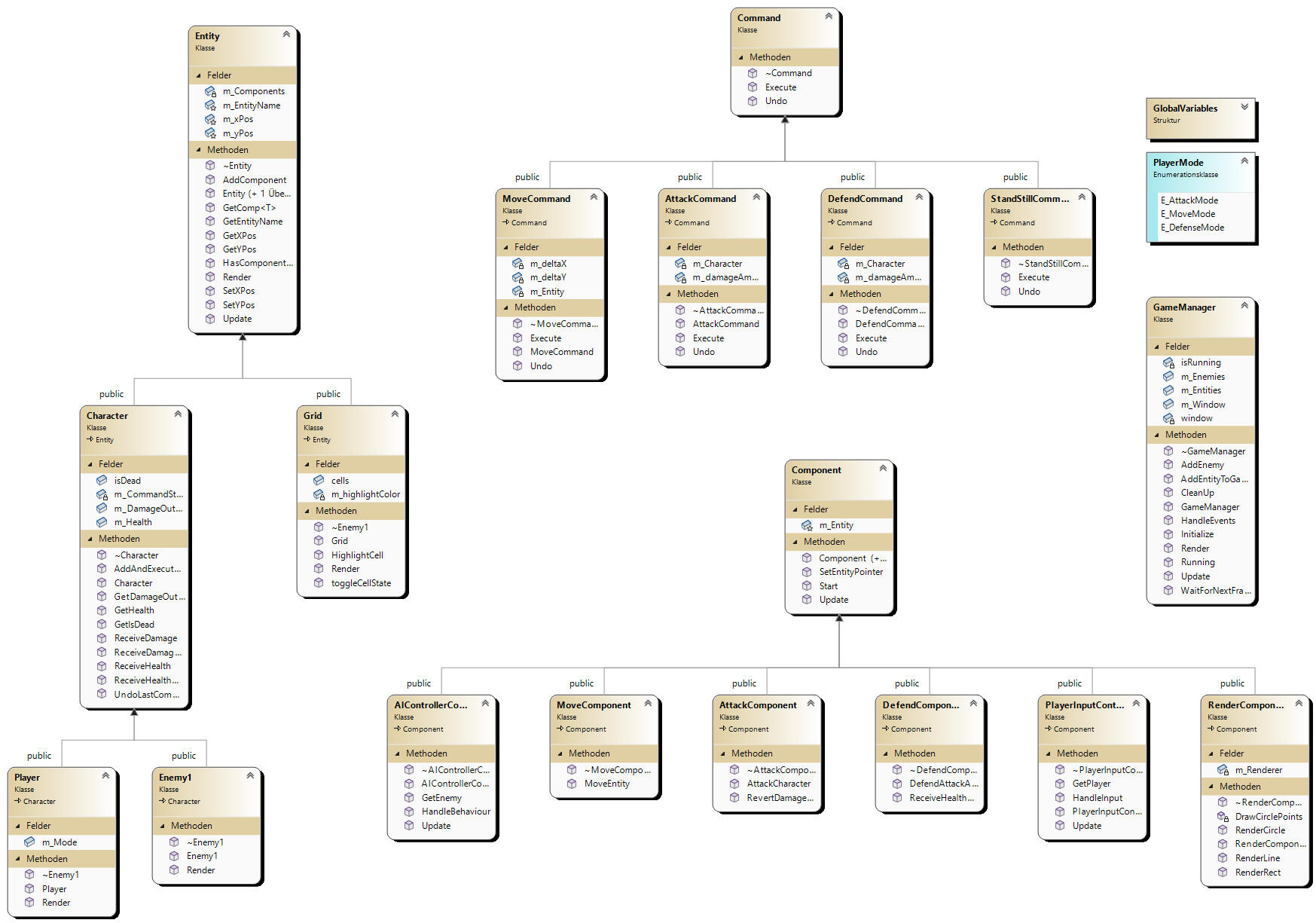
Hologram Shader
My goal for this project was to create a hologram shader with the following properties:
Glitch effect
Dynamic, moving edges
Radiant, pulsating borders
Interchangeable scan textures moving across the hologram
Dissolve effect
Primarily, I utilized noise functions for the most part, which aligns with the essence of the project, as glitches and additional variability play a crucial role in a hologram shader.
Glitch Effect
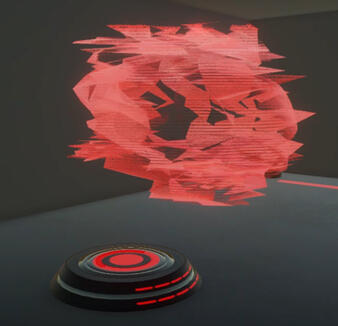
For this feature, I employed a noise function in conjunction with a varying x-offset to control a transformation shift in the x-direction via a code script.
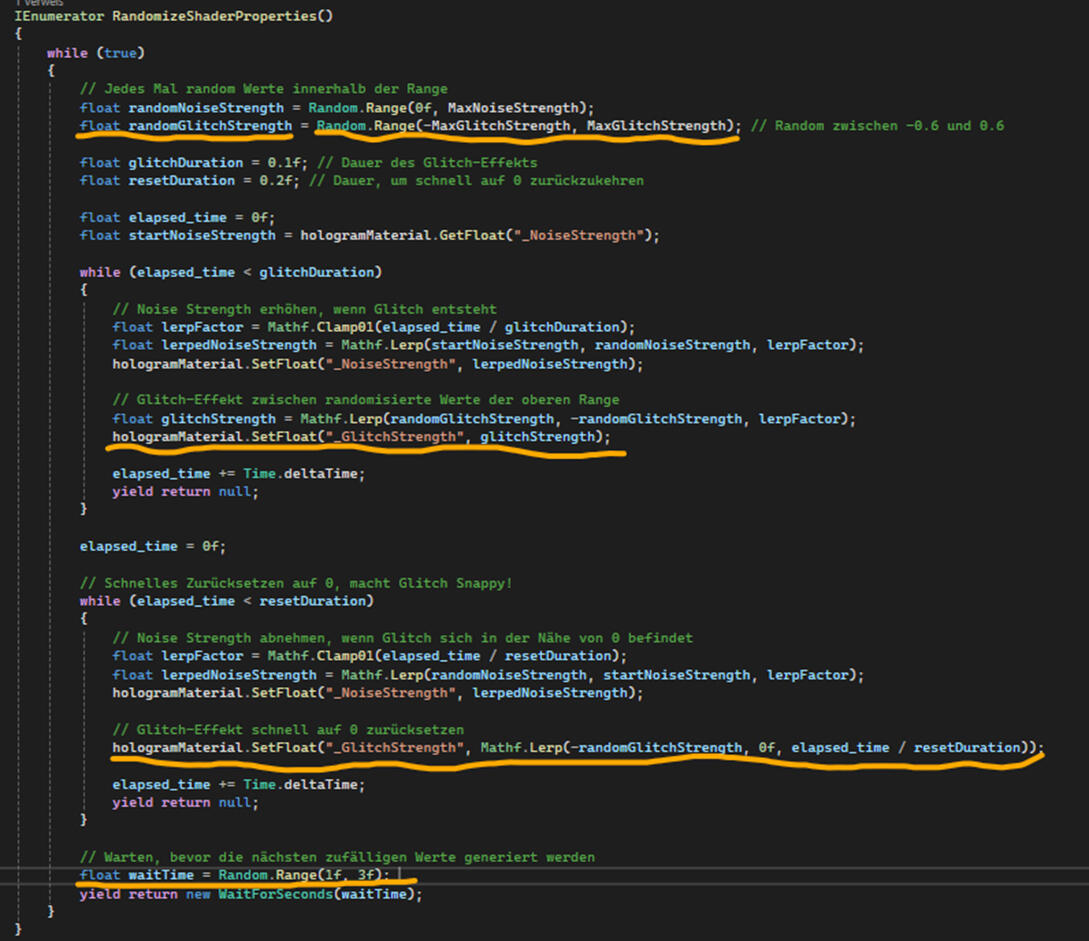
Dynamic, Moving Edges
Here, I utilized the sine function in combination with the simple noise function to achieve a consistent motion with a touch of noise.
Radiant, Pulsating Borders
To achieve the emission effects, I incorporated a basic Fresnel effect and infused it with a pulsating attribute, modulated by noise for added dynamism.
Interchangeable scan textures moving across the hologram
First, I utilize a Time node and multiply it by a value. This allows me to adjust the speed of the process via the property. Subsequently, this value is added to the vertical world position and stored in the Y component of a Vector2. This enables me to overlay a hologram scanline texture onto the hologram object, creating a dynamic effect.
Dissolve Effect
I toyed with the idea of disintegrating the hologram itself, exploring an approach where the object position is multiplied by a value. This method aims to converge all vertices towards the object's pivot point, simulating a shrinking effect akin to a black hole. However, after experimenting with and implementing this approach, I found the results to be unsatisfactory, prompting me to explore alternative methods.
In the new approach, the dissolve effect is achieved using Smoothstep and gradient noise, and it is subsequently controlled through linear interpolation within a script. This process is demonstrated in the video above on the page.
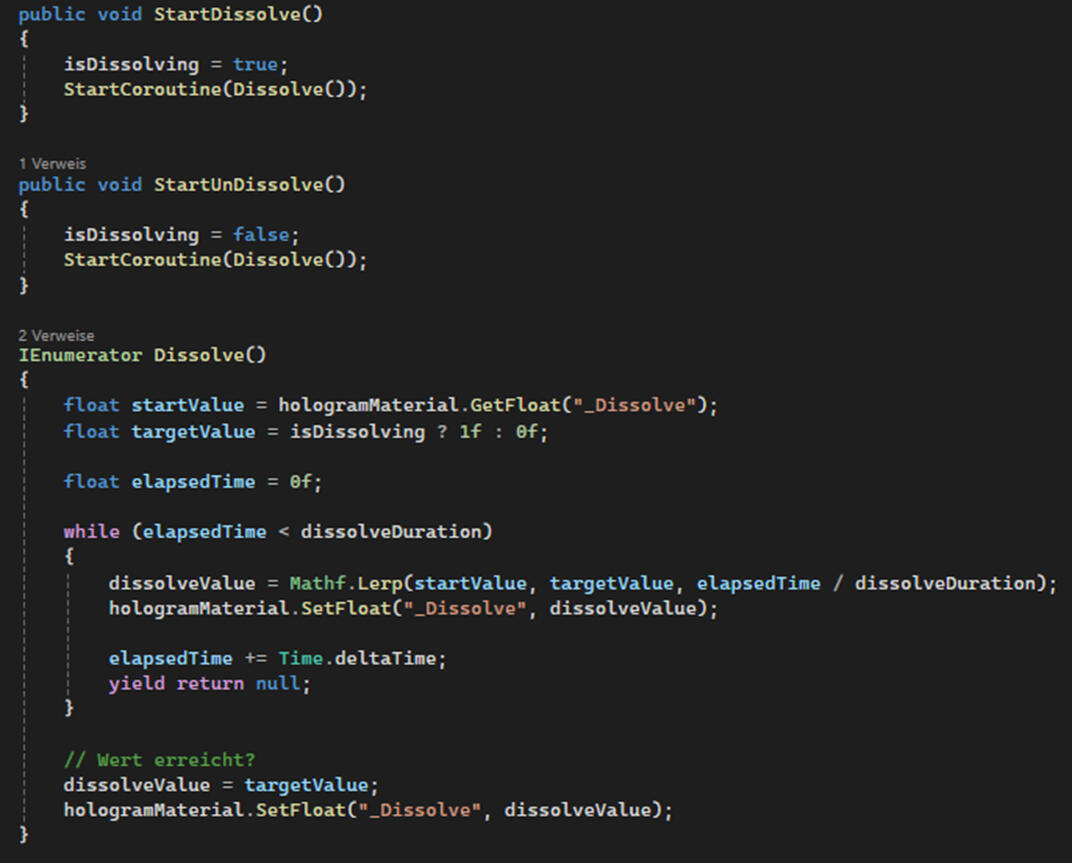
The project was initially implemented using HLSL and later with the assistance of Shader Graph.
Card Game KI (MinMax Algorithm)
In this project, I attempted to design a card game along with an AI that makes decisions using the MinMax algorithm.
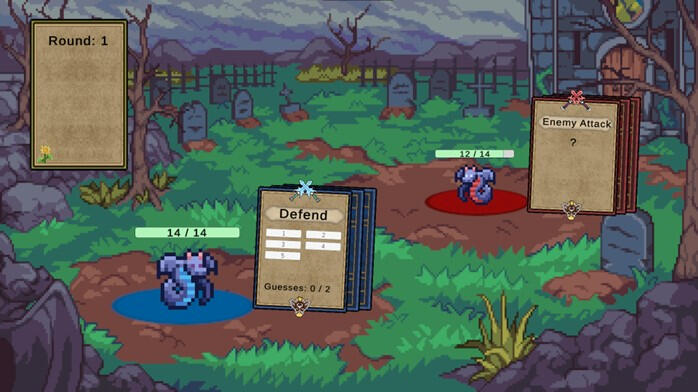
Game Rules
Each player starts with the same number of cards.
Every card has a Defense Value and an Attack Value.
The Defense Value determines how many attempts the cardholder can make to block an opponent's attack.
The Attack Value defines the strength of the card's attack. The player selects a number between 1 and the card's Attack Value to execute their attack.
GameFlow: (-> PlayerAttack -> EnemyDefend -> EnemyAttack -> PlayerDefend ->) over 4 Rounds
At the start of each round, both players set their Attack and Defense values.
Programming
GameManager
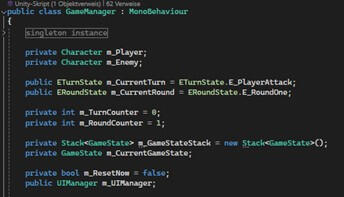
The GameManager itself uses the Singleton Pattern. It manages the round and turn counters to transition between states accordingly. The GameState stack is utilized by the MinMax algorithm to manage the game states during the simulation. The m_CurrentGameStack updates the current game state in real-time.
Character Class
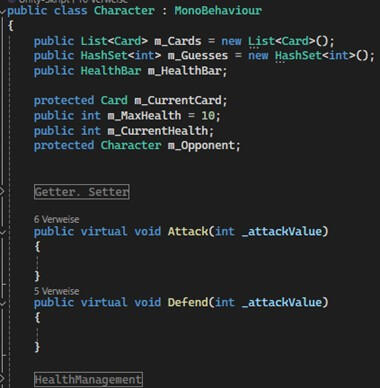
The Character class serves as the foundation for the Player and Enemy classes. It stores components such as the cards, defensive guesses, and other attributes like the opponent itself or health points. The subclasses can utilize and manage these components for their specific needs. The defensive guesses are implemented as a HashSet<int> to ensure that no guess is repeated within a single turn.
GameState
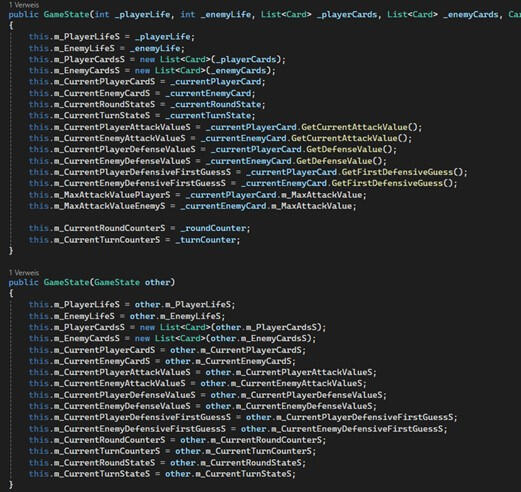
A "Deep Copy" needs to be created here to ensure that the GameStates do not influence or modify each other.
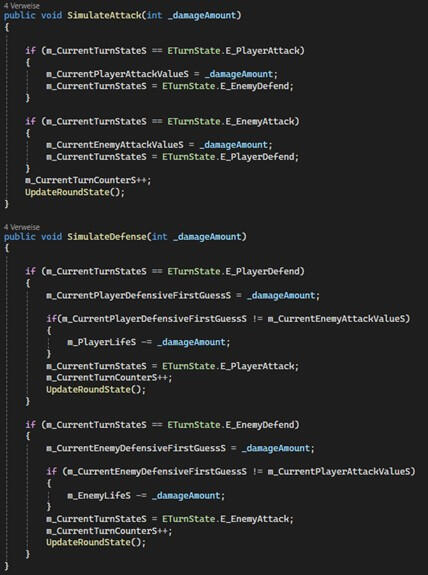
Just like in the real game, the Attack and Defend functions are used in the simulated GameState. The actual values of the player cards are not altered; only the simulated values are changed. Additionally, the TurnStates determine who will attack and who must defend (player or AI).
MinMax KI

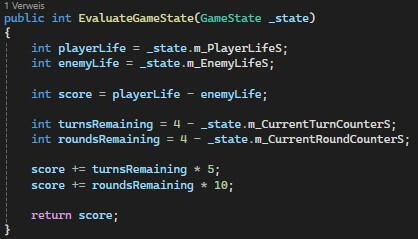
The MinMax algorithm always uses an evaluation function to assess each GameState and return a score. This score is used to determine whether an action was good or bad, guiding the AI's next move.

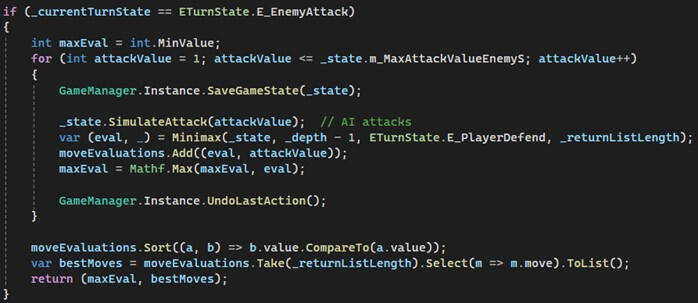
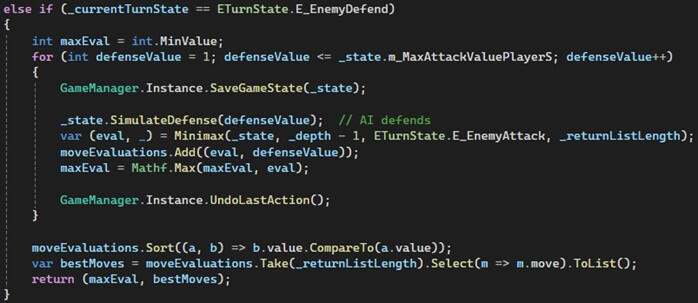
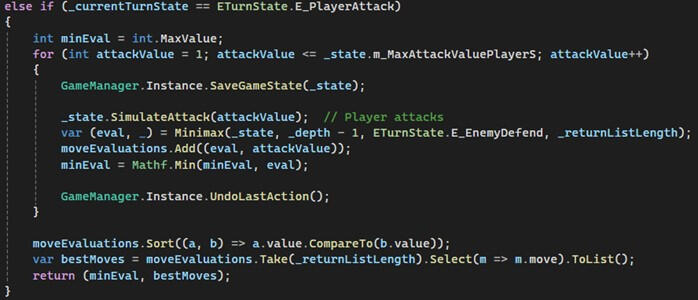
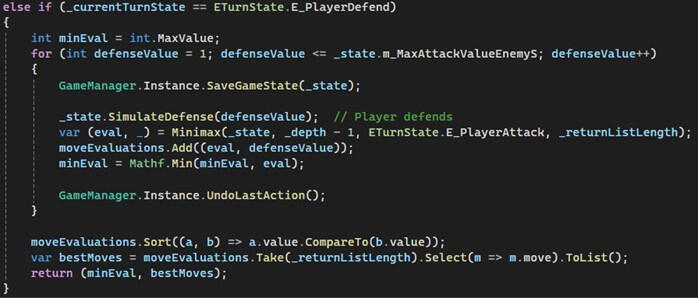
ASCII Game Engine
// Project will start in June 2025Together with a fellow student, we will develop a small ASCII game engine as part of our upcoming project work.
Encrypted Haptic Messaging
I am currently designing a system consisting of a backend server and an Android app that allows users to manage and send custom vibration patterns.Within the app, users can create and manage vibration patterns. With the press of a button, a custom vibration pattern can be encrypted and sent to another phoneThe goal is to send short messages, similar to Morse code. With the use of distinct vibration patterns, the message no longer needs to be read. — it can simply be felt.In the near future, I plan to expand the app to make it compatible with certain smartwatches.
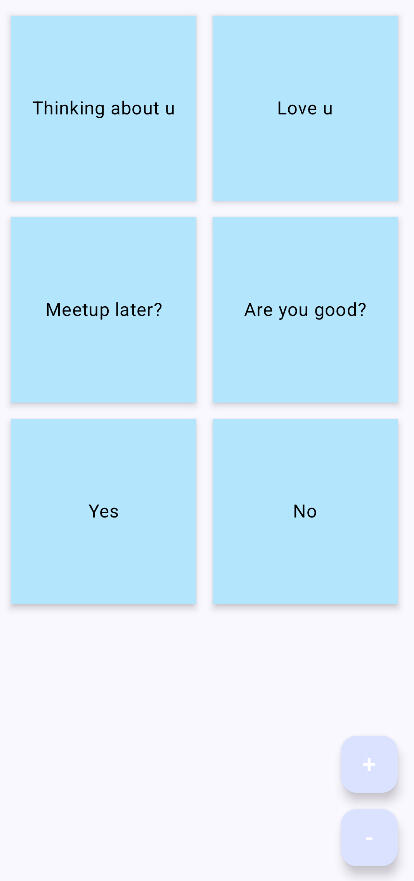
The server environment is hosted on my Raspberry Pi, acting as an interface between my Android app and Google Firebase.(Firebase is used to efficiently receive push notifications with minimal resource consumption.)
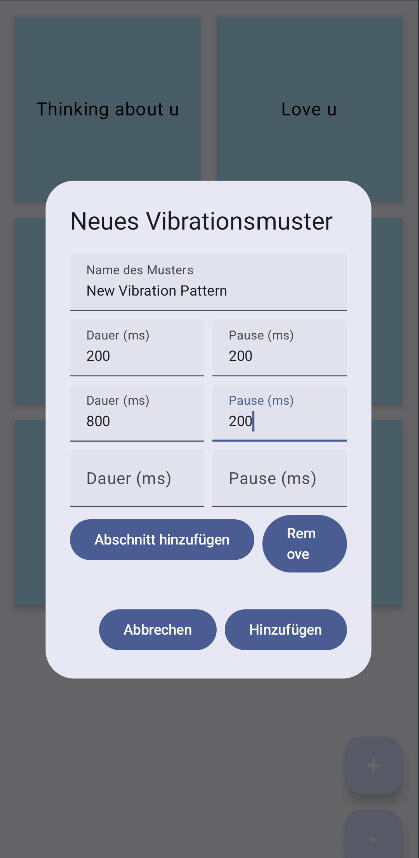
With this project, I also aim to learn the security principles of managing a public server.For long-term server operation, I may consider a cloud-based solution, but I don't want to miss out on the learning experience.
Stickbound
In Stickbound, you take on the role of a puppet bound to a stick, which has been mysteriously brought to life. Awakening deep within a subterranean cave network, your journey unfolds towards the surface, yearning for the warmth of sunlight once more. Alongside mastering the art of grappling hook maneuvers and dash mechanics, you confront various adversaries. Can you prevail and secure your freedom? The game offers a 2D action platformer experience, integrating traditional gameplay with the innovative addition of a grappling hook.
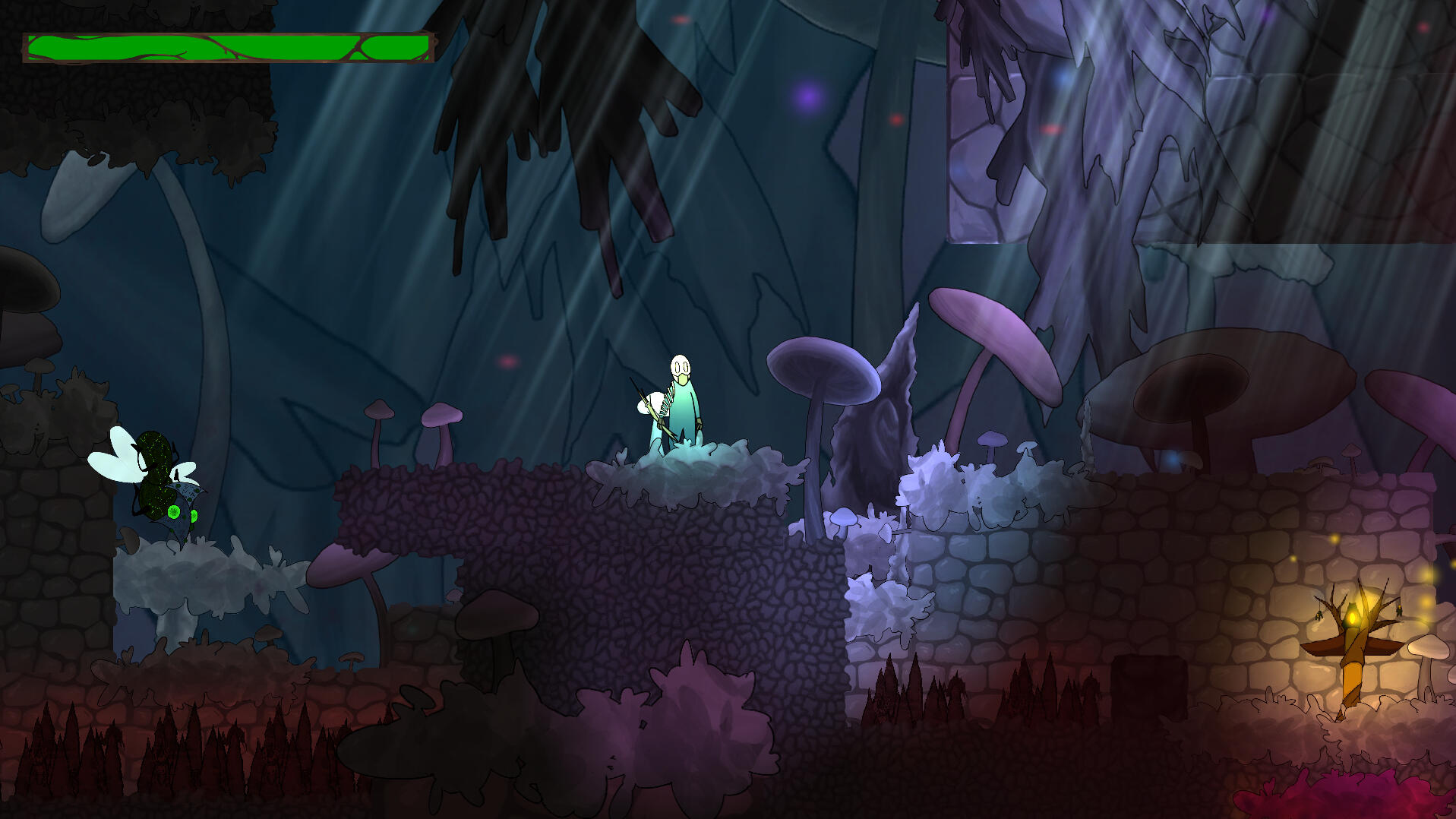
Role: Solo-Programmer (+ parts of Game Design, Level Design)
Tools: Unity (C#), GitHub, Trello
Core-Team: Six Students
Time Span: 1,5 Months
Programming
I was responsible for implementing the movement mechanics, developing a camera system, and designing a finite state machine model for the enemies, while also handling the complete coding of the game.
Camera Control
For the camera control, I utilized the Cinemachine extension from Unity. Typically, the player is simply dragged into the Follow slot of the Cinemachine Virtual Camera in the Inspector to align the camera with the player. However, rapid changes in direction and camera offset can lead to significant issues. Therefore, a lerp is simulated by having the Cinemachine follow a PlayerFollowObject, and this object, in turn, tracks the player with a specific delay. This results in smooth camera control.
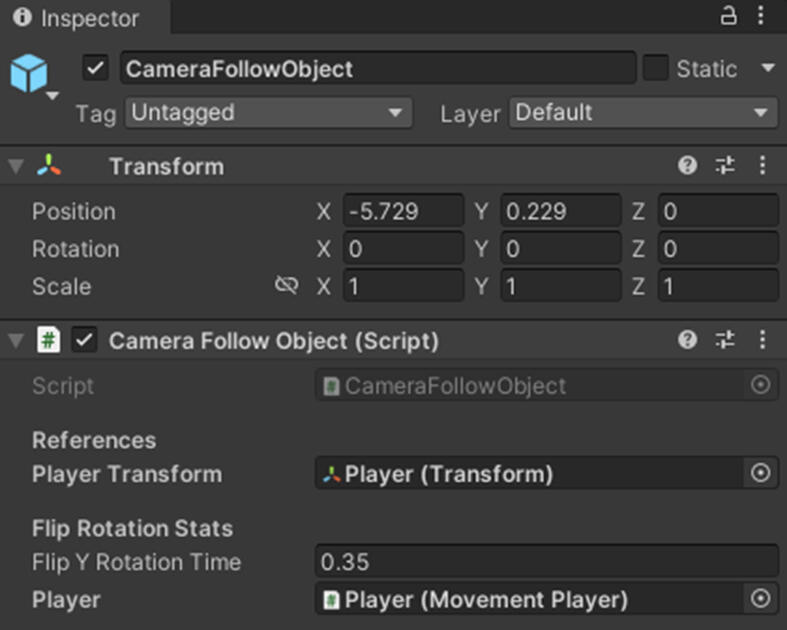
Furthermore, there is a Camera Manager in place to oversee all Cinemachine Virtual Cameras integrated within the game.
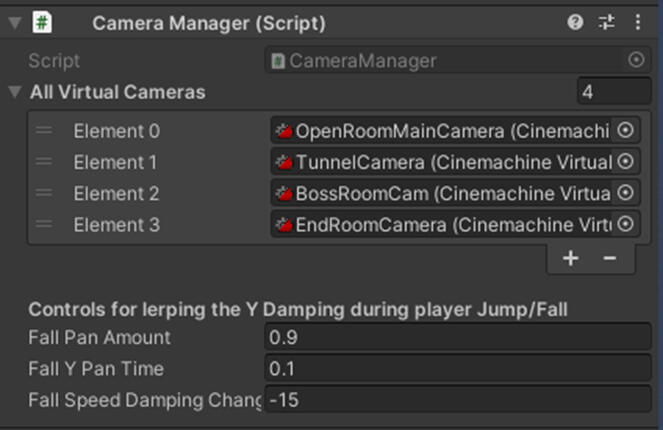
The final aspect of the camera in this game is a Scriptable Object that can access the Pan and Swap methods of the Camera Manager. In this setup, a Collider with a Trigger can be easily dragged into the scene using a Prefab. Then, in the Inspector, one can simply adjust the camera behavior at specific locations based on the chosen options.
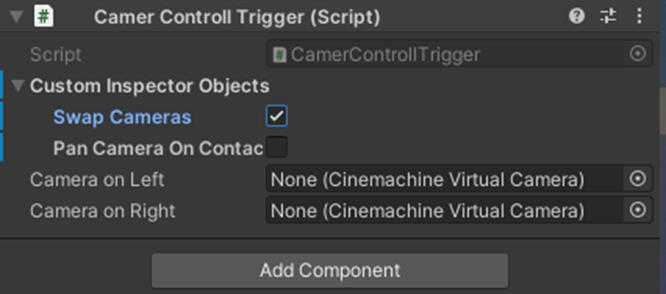
Player Movement
I've finely tuned the player movement to match the dynamic feel of the game. In addition to the basic mechanics of a 2D platformer, I've incorporated a Grappling Hook mechanic (through Raycasting) alongside features like Coyote Timers, Variable Jump Height, Jump Buffering, Clamped Fall Speed, and many others.
Finite State Machine Model for Enemies
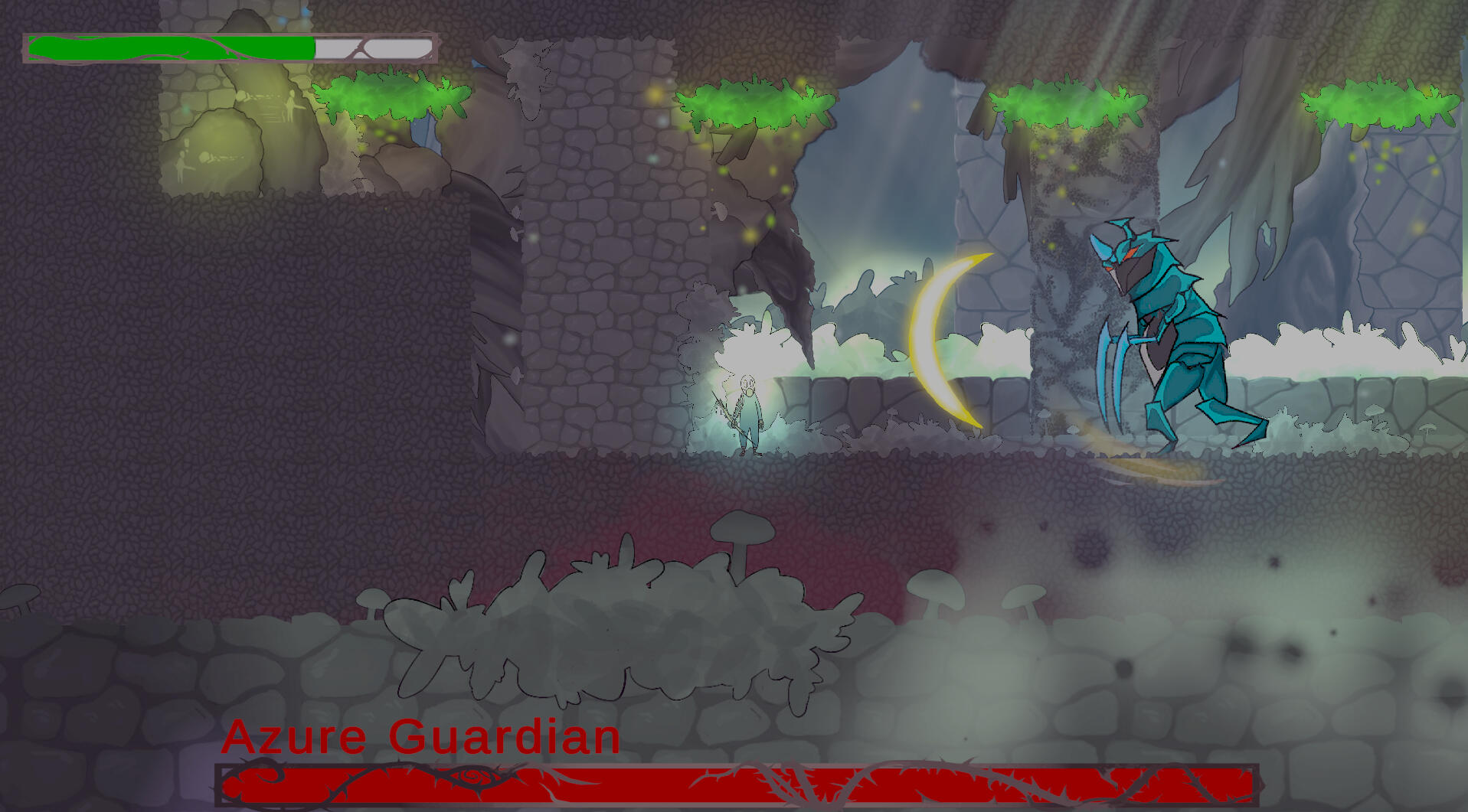
Basierend auf Vererbung wird eine
Entity Klasse erstellt, von denen alle zukünftigen Gegner erben können. Es müssen außerdem generelle States festgelegt werden,
die dann in den spezifischen Gegner Typen genauer definiert werden.
Setup:
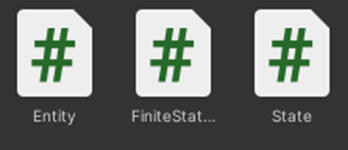
Entity serves as the base enemy script, while the other two are responsible for state transitions. Subsequently, a script is created for each state, each of which inherits from State.

For each state, a data script is created.

Using this template, one can easily set up the enemy and expand upon it by adding new states and transitions.
Example Enemy:
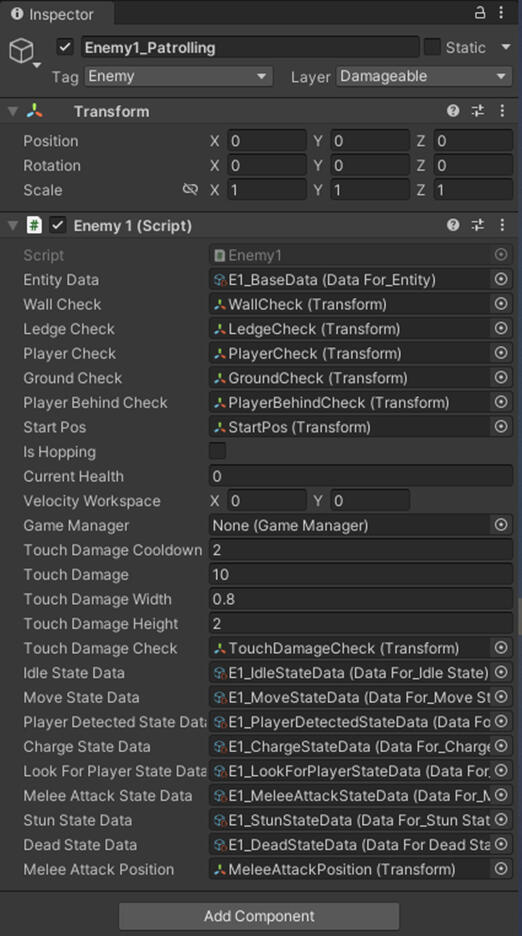
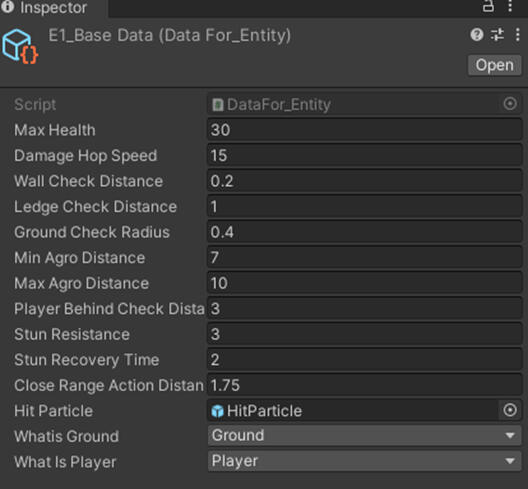
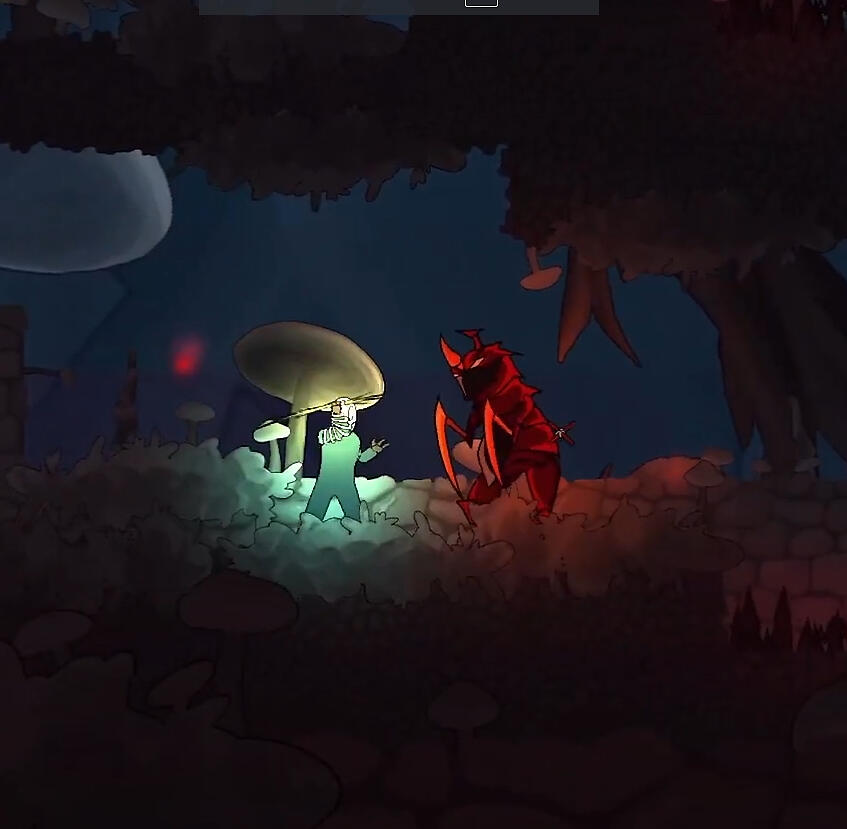
This type of enemies served as the foundational patrolling enemy in the game.
ExhibitA
Exhibit A is a first person detective game in which the player has to find out who killed a painting and sabotaged a new AI painting by examining pictures, eavesdropping on conversations and much more.
The museum combines traditional, hand-painted and digital works of art in one environment.
The game was nominated for the Deutscher Computerspielpreis Nachwuchspreis (German Computer Game Award, Newcomer Award) in the Best Prototype category in 2025!!
The game was created during my third semester at Mediadesign Hochschule in Munich.
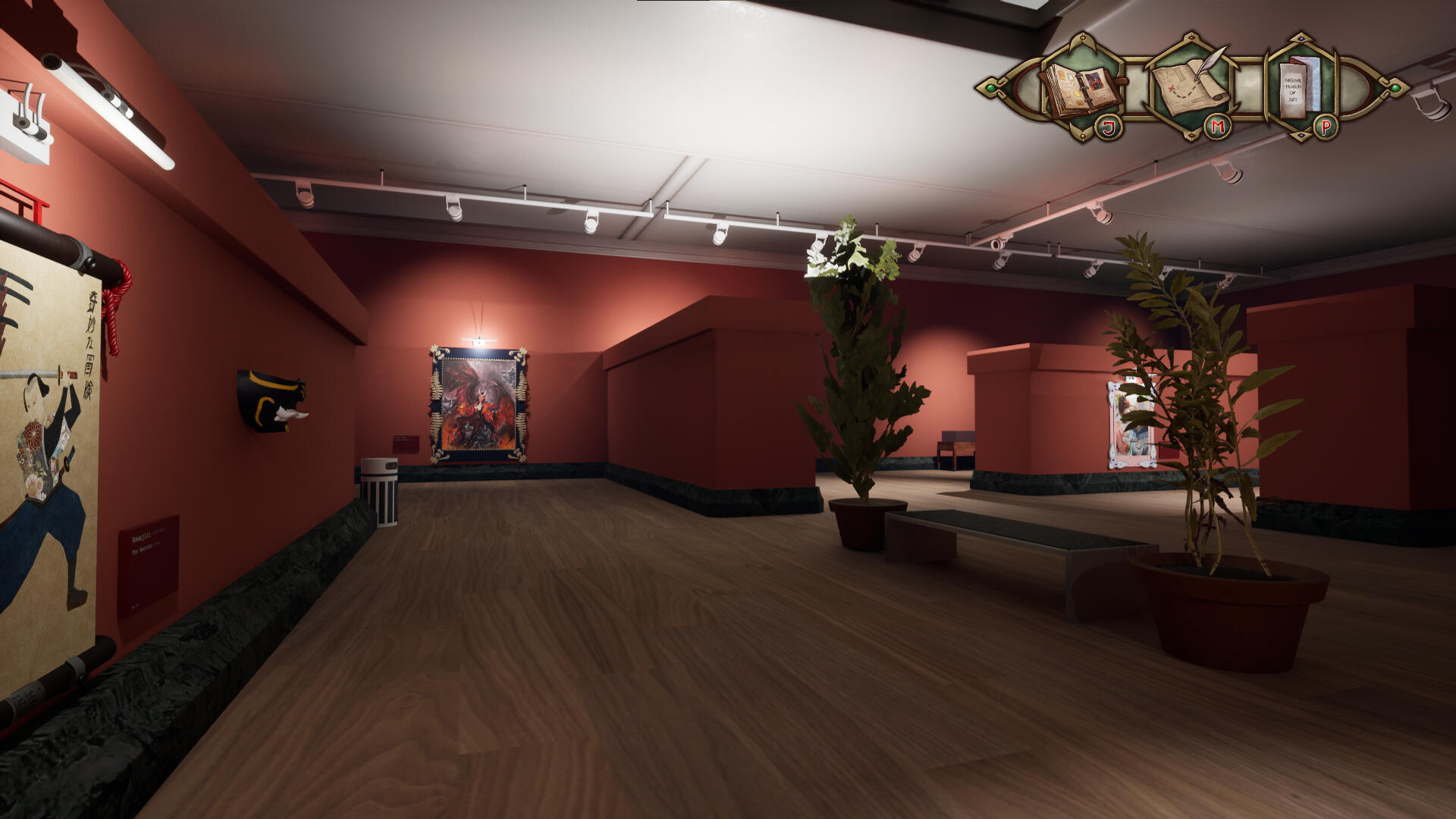
Role: Solo-Programmer
Tools: Unreal Engine 5, Perforce, Jira
Core-Team: Six Students
Time Span: 1,5 Months
Programming
I was responsible for implementing the inspect/hide mechanic, developing a dialogue system, and designing a drawing mechanic in order to make a responsive map, while also handling the complete coding of the game.
Map System
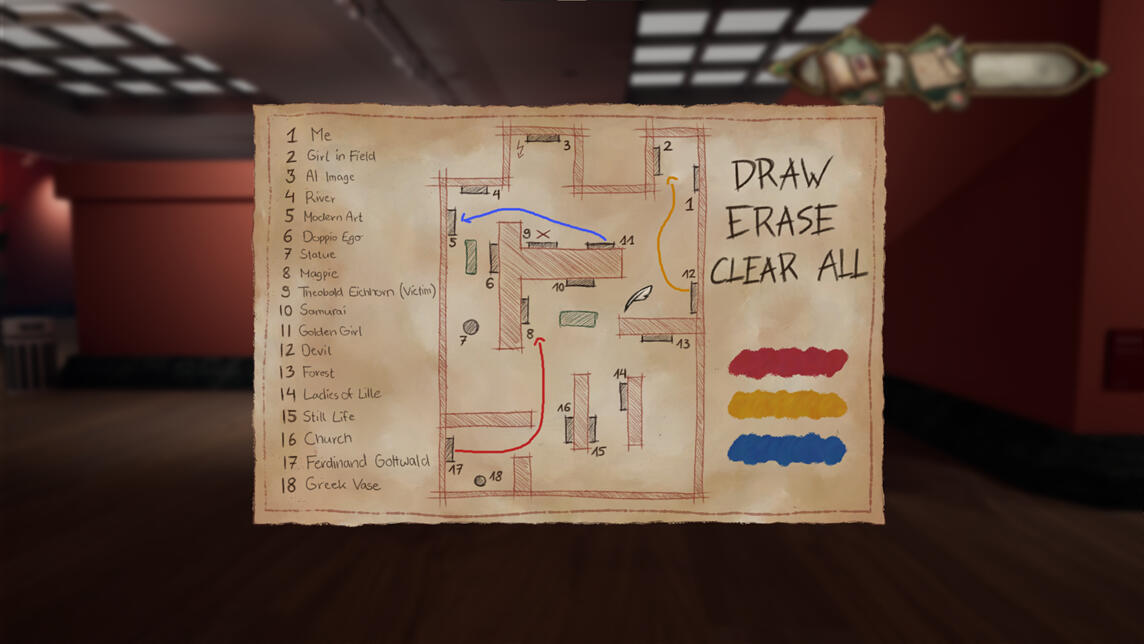
I first created an Enum capable of storing 3 colors. Subsequently, I developed a data structure that stores a 2D vector array, as well as the Color Enum. The Vector2D array represents a line, and within the OuterContainerArray, all drawn lines can be stored. All lines inside this container array are then updated accordingly through the actions of erase, draw, or clear all.
Inspect System
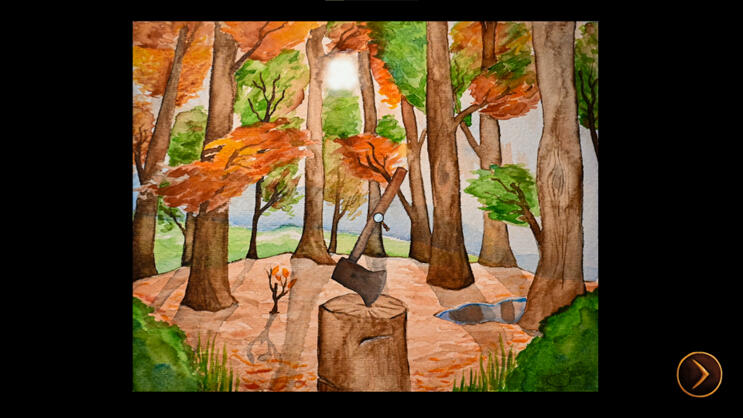
For this mechanic, the camera transitions from the spot it's currently on using a fade-to-black effect and motion manipulated through linear interpolation. It then displays a 2D image that allows interaction, enabling objects to be taken from the image.
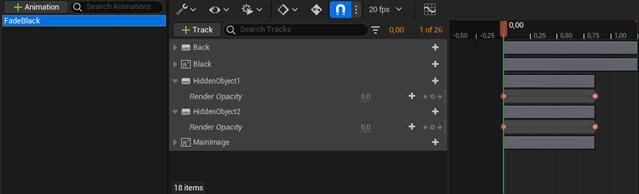
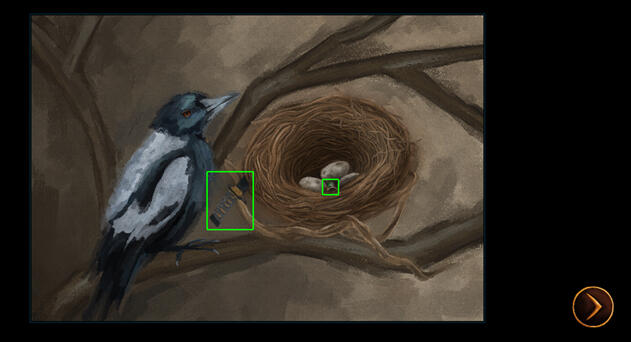
In the Details Panel, the most important public variables are CameraToFind and MaterialToChangeTo. The camera is used for blending, and the material is utilized to ensure that objects taken from the inspect widget are also altered in the scene.
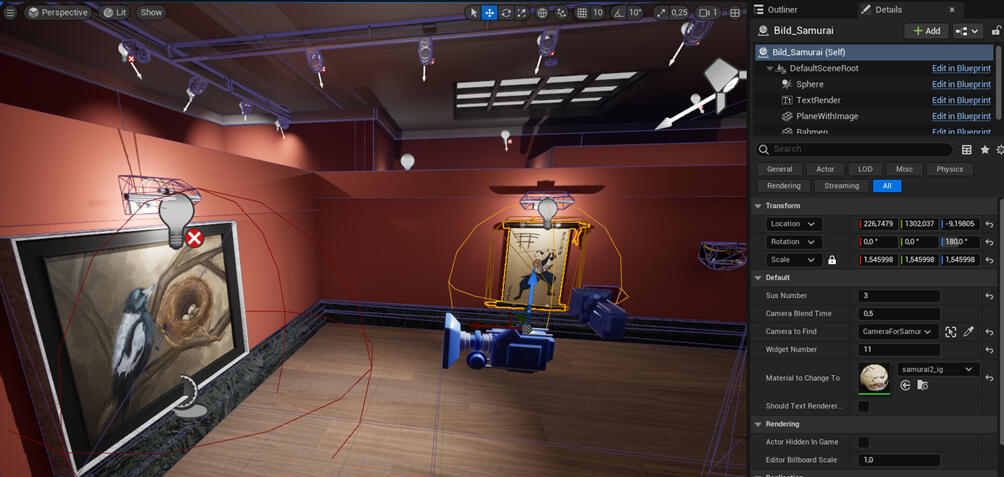
The approach used for the Hide System was simply repurposed, replacing the fade-to-black effect with a dialogue window to eavesdrop on specific images
Fang-tastic Heist
Fang-tastic Heist is a 2D Point-and-Click Adventure game, in which a goblin and his friends have decided to sneak into a vampire castle and steal it's riches. During their heist those friends get captured and now it is your job to use your wits and find a way to rescue your crew.
Role: Tech-Lead
Tools: Unity, C#, Jira
Core-Team: Six Students
Time Span: 1,5 Months (Production)
// Soon will be updated (Game already finished)
Addictive Factors in MMORPGs:
A Case Study of World of Warcraft (term paper)
The paper dives into why MMORPGs, with World of Warcraft as the prime example, can become so addicting. It explores the allure of this genre and what keeps players engaged. Starting with definitions of addiction and dependency, it then explores the current research on internet and gaming addiction. The MMORPG genre itself gets a close examination. Using World of Warcraft as a case study, the paper breaks down its components and structures to uncover what makes it so compelling.
The term paper is currently only available in german.